Manipulating time and date is infamously challenging. When developers face the complexities of time zone regulations, leap seconds, and variations in locale-specific formatting, it’s prudent for them to lean on well-regarded time and date manipulation libraries. However, without a deep understanding of their mechanics, it remains alarmingly simple to introduce a myriad of subtle errors. In this piece, I aim to guide you through a clear thought process regarding date and time management, while offering some best practices designed to steer you clear of the pitfalls associated with handling date/time data. We will delve into essential concepts vital for accurately handling date and time information, discuss formats that facilitate the efficient storage and API transfer of DateTime values, and more.
Mastering Timestamp Retrieval and Formatting in JavaScript: A Comprehensive Guide
In JavaScript, obtaining the current timestamp is pivotal for various applications, from time-sensitive operations to data tracking and synchronization. Fortunately, there are several methods to retrieve this essential piece of information effortlessly.
Using Date.now()
One of the simplest and most direct ways to fetch the current timestamp is by utilizing the Date.now() method. This method seamlessly provides the number of milliseconds elapsed since January 1, 1970, 00:00:00 UTC, commonly referred to as the “epoch.”
Implementation Example:
const timestamp = Date.now();
console.log(timestamp); // prints the current timestamp
Advantages of Date.now():
- Simplicity: It offers a straightforward approach with minimal code required;
- Efficiency: Date.now() is highly efficient in fetching the current timestamp without creating additional objects;
- Accuracy: The returned value precisely represents the current time in milliseconds, ensuring accuracy in time-sensitive applications.
Utilizing new Date().getTime()
Alternatively, developers can opt for creating a new Date object and invoking the getTime() method to acquire the timestamp. Remarkably, this approach yields the same result as Date.now(), providing flexibility in implementation.
Implementation Example:
const date = new Date();
const timestamp = date.getTime();
console.log(timestamp); // prints the current timestamp
Benefits of new Date().getTime():
- Compatibility: It ensures compatibility with older JavaScript versions where Date.now() might not be available;
- Versatility: Developers can leverage the new Date() object for additional date and time manipulations if necessary;
- Readable Code: While slightly more verbose, this method offers clarity in code readability, aiding in maintenance and collaboration.
Timestamp Formatting Considerations
It’s essential to note that the timestamp returned by both Date.now() and getTime() is a large numerical value, representing milliseconds since the epoch. To present this information in a human-readable format, further processing is often necessary.
Formatting Options:
- toString() Method: JavaScript’s built-in toString() method can be utilized to convert the timestamp to a string representation;
- Moment.js Library: For comprehensive date and time formatting capabilities, integrating libraries like Moment.js proves highly beneficial. Moment.js offers extensive functionality for parsing, validating, manipulating, and formatting dates and times.
Implementation Insights:
- Precision vs. Readability: Choose formatting options based on the specific requirements of your application. While precision is crucial for computational tasks, readability is paramount for user-facing interfaces;
- Localization: Consider incorporating localization features to cater to diverse audiences with varying date and time preferences.
Obtaining the Current Timestamp with Moment.js
Moment.js is a versatile library that makes working with dates and times in JavaScript a breeze. If you’re looking to get the current timestamp and format it as a date and time string in Coordinated Universal Time (UTC), Moment.js provides a straightforward solution.
Using Moment.js in Node.js:
To get started, you’ll first need to install Moment.js in your Node.js project. You can do this using npm or yarn:
npm install moment
Once Moment.js is installed, you can require it in your Node.js application:
const moment = require('moment');
With Moment.js imported, you can now obtain the current timestamp in UTC and format it as a string:
const timestamp = moment().utc().format();
console.log(timestamp); // prints the current timestamp in UTC as a string
In the above code:
- We use moment() to create a new moment object representing the current date and time;
- .utc() is used to switch the moment object to UTC mode, ensuring consistency across different time zones;
- .format() converts the moment object to a string representation based on the specified format.
Expanding on Timestamp Generation:
While the example above demonstrates a simple use case, there are numerous scenarios where you might need to manipulate timestamps in your JavaScript applications. Here are some additional tips and insights:
- Custom Formatting: Moment.js offers extensive formatting options, allowing you to tailor the timestamp output to your specific requirements. You can specify date formats, include time zones, and even display relative time (e.g., “2 hours ago”);
- Time Zone Handling: Dealing with time zones can be complex, but Moment.js simplifies the process by providing built-in support for handling time zone conversions and offsets. Whether you’re working with local or international users, Moment.js has you covered;
- Date Arithmetic: Need to perform calculations with dates and times? Moment.js makes it easy to add or subtract days, months, years, hours, minutes, or seconds from a given timestamp. This functionality is particularly useful for tasks like scheduling events or calculating durations;
- Localization: If your application targets users from different regions, you can use Moment.js to localize date and time formats according to their preferred language and cultural conventions. This ensures a seamless user experience regardless of geographical location.
Choosing the Right Approach:
While Moment.js is a powerful tool for working with dates and times, it’s essential to consider your project’s specific requirements before deciding on a timestamp generation method. Here are some factors to keep in mind:
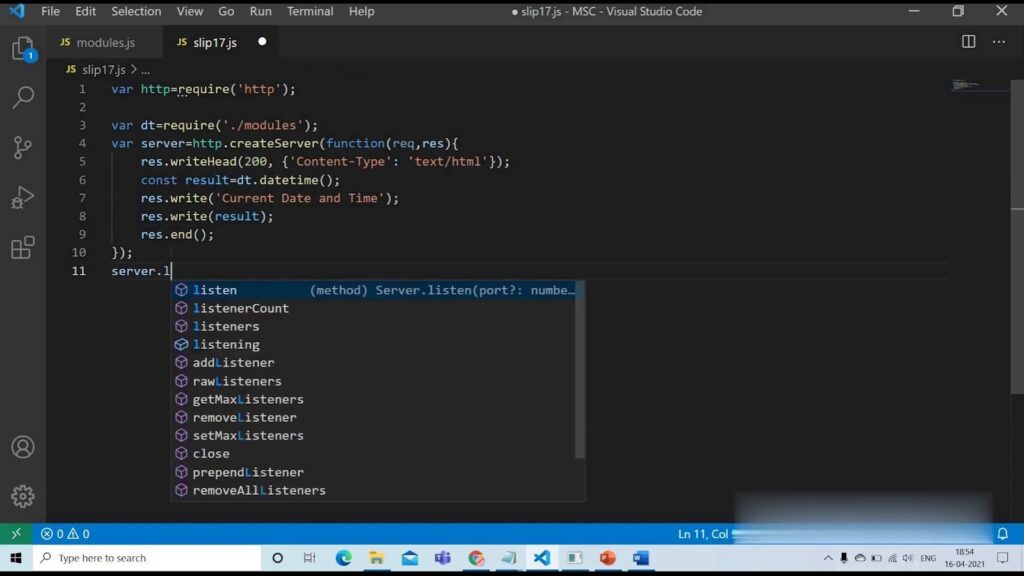
- Complexity: If your timestamp needs are relatively straightforward, using the built-in Date object and its methods might suffice. However, for more complex scenarios involving time zone conversions, formatting, or arithmetic, Moment.js offers a more robust solution;
- Dependencies: While Moment.js provides extensive functionality, it adds an additional dependency to your project. If you’re concerned about bundle size or performance, consider whether the features offered by Moment.js justify the overhead of including it in your application;
- Future Compatibility: As of this writing, Moment.js remains a popular choice for date and time manipulation in JavaScript. However, it’s essential to stay informed about updates and alternatives within the JavaScript ecosystem. Keep an eye on emerging libraries and standards that may offer more efficient or modern solutions.
Timestamp in UTC (Coordinated Universal Time)
Calculating timestamps in Coordinated Universal Time (UTC) is crucial for various applications, especially those reliant on synchronized timekeeping across different time zones. Whether you’re tracking events, managing distributed systems, or recording data, understanding how to generate timestamps in UTC ensures consistency and accuracy. Here’s a comprehensive guide to mastering UTC timestamps and their applications:
Importance of UTC Timestamps:
- UTC serves as the global standard for timekeeping, unaffected by daylight saving time or regional variations;
- Timestamps in UTC facilitate coordination across international boundaries, avoiding confusion caused by different time zones;
- Many computer systems and protocols rely on UTC for consistency in data exchange and synchronization.
Generating UTC Timestamps:
- Utilize JavaScript’s Date object in conjunction with methods like Date.UTC() or getTime() to calculate UTC timestamps;
- The Date.UTC() method constructs a date object using UTC time zone values, returning milliseconds since January 1, 1970;
- Alternatively, calling getTime() on a Date object created with Date.UTC() provides the current timestamp in UTC.
Formatting UTC Timestamps:
- Convert UTC timestamps into human-readable date and time strings using methods like toUTCString() or libraries such as moment.js;
- Custom formatting options allow displaying timestamps in various formats, accommodating diverse application requirements.
Applications and Use Cases:
- Distributed Systems: Timestamping events and transactions in UTC ensures consistency and facilitates chronological ordering in distributed systems;
- Logging and Debugging: Incorporating UTC timestamps in logs aids in troubleshooting by providing a standardized timeline of system activities;
- Data Recording: Recording data with UTC timestamps simplifies data aggregation and analysis, particularly in global contexts or when collaborating across time zones.
Best Practices:
- Always use UTC timestamps for system operations, especially in distributed or international environments;
- Ensure system clocks are synchronized with UTC to avoid discrepancies in timestamp generation;
- Regularly update timestamp handling mechanisms to accommodate changes in timekeeping standards or software updates.
Conclusion
In conclusion, mastering the intricacies of date and time manipulation is a crucial skill for developers, given the potential for errors that can arise from misunderstandings or misuse of time zones, leap seconds, and locale-specific formatting. By adopting best practices and leveraging the capabilities of reputable libraries, developers can significantly reduce the risk of bugs and ensure their applications handle date and time data accurately and efficiently. This exploration of key concepts, storage formats, and transfer methods for DateTime values aims to equip you with the knowledge and tools needed to navigate the complexities of date and time manipulation with confidence, avoiding the common pitfalls that lead to the dreaded date/time management challenges.