Real-time flight tracking has become a valuable tool for aviation enthusiasts, professionals, and anyone interested in monitoring flights across the globe. Sites like this website provide comprehensive, live tracking of aircraft, helping users follow flight paths, check arrivals and departures, and stay updated on air traffic. If you’re interested in building your own real-time flight tracking system, Node.js offers a powerful platform to create such an application, combining real-time data processing and seamless server-side integration.
In this guide, we’ll walk through how to create a real-time flight tracking server using Node.js. The goal is to set up a server that can gather flight data from an external API and display it on a web interface in real-time. This kind of server can be used to monitor global flight activities or focus on specific regions or airlines, depending on your needs and the data source you choose to integrate.
Understanding Flight Data Sources
Flight tracking systems work by collecting data from various sources, including transponders, radars, and satellites. Aircraft typically broadcast information such as their current location, altitude, speed, and heading via transponders that communicate with ground stations or satellite systems. These ground stations capture the broadcast data and send it to centralized databases, which are then accessed by flight tracking services.
One of the most popular technologies for tracking flights is ADS-B (Automatic Dependent Surveillance-Broadcast), which allows aircraft to continuously send out their positional data to any nearby receiving stations.
The Benefits of Using Node.js for Real-Time Applications
Node.js is particularly well-suited for real-time applications like flight tracking because of its event-driven architecture. Unlike traditional server environments that rely on creating new threads for each request, Node.js uses an asynchronous, non-blocking I/O model that can handle thousands of simultaneous connections. This feature is critical for real-time applications where data needs to be updated and served to multiple clients simultaneously without delay.
For a real-time flight tracking system, Node.js allows you to:
- Fetch live flight data from external APIs and process it quickly.
- Use WebSockets to push real-time updates to connected clients.
- Build a fast and responsive web server that can manage multiple requests and connections at once.
By using Node.js, you can streamline the process of gathering and serving flight data to users, ensuring they receive updates as quickly as possible.
Setting Up Your Node.js Server
To begin, you’ll need to install Node.js and set up a basic Node.js project. Once installed, the next step is to build a web server that can handle requests and fetch flight data from an external API. Flight tracking APIs typically provide endpoints where you can query real-time flight information based on various parameters such as flight number, airport code, or geographic location.
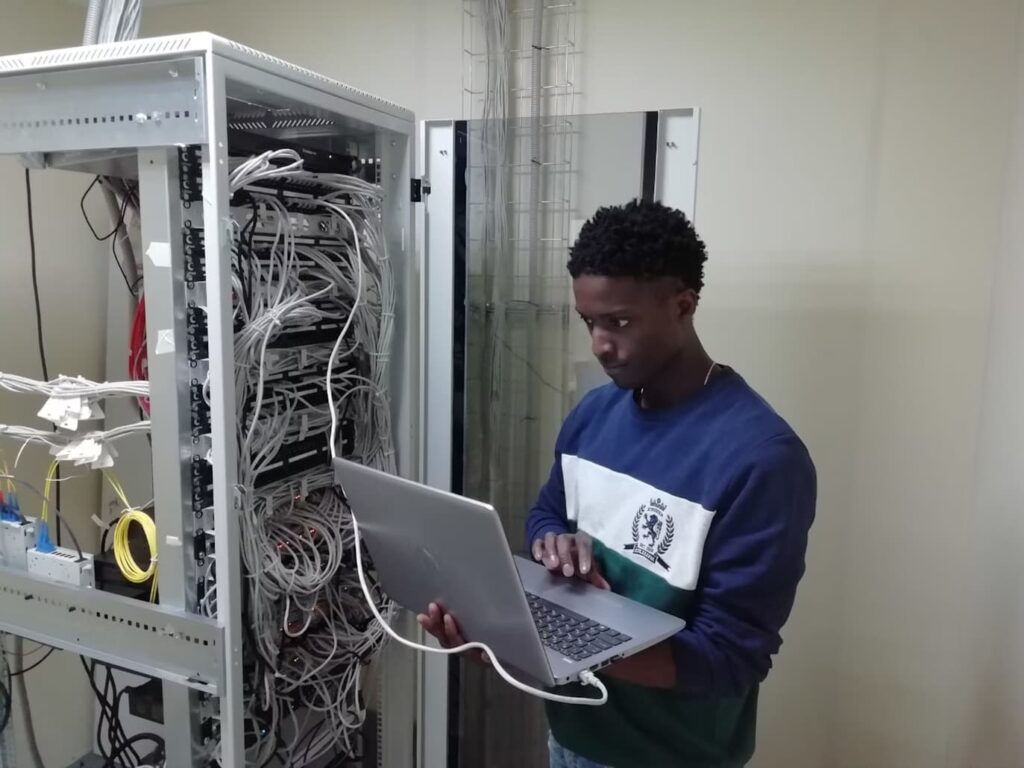
When building your server, you should aim to:
- Create a web server that listens for incoming connections and serves an interface where users can view flight data.
- Use an HTTP client to fetch data from an API, which could be a free or paid service depending on the scope of your project. Some APIs offer a limited number of requests per day, while others might offer higher access tiers for premium users.
- Implement WebSockets to send real-time updates to connected clients whenever new flight data is available.
A well-designed flight tracking server will continuously fetch updated data from the external API at intervals (e.g., every 10 seconds) and push that data to users without them needing to refresh the page manually. This can be accomplished through server-side logic that checks for new flight information and immediately relays it to clients.
Real-Time Communication with WebSockets
To ensure that your server is capable of delivering real-time flight data, you can use WebSockets, which allow for continuous, two-way communication between the server and clients. Unlike traditional HTTP requests, which require the client to poll the server for updates, WebSockets keep the connection open, allowing the server to push new data to clients as soon as it’s available.
In a real-time flight tracking system, WebSockets are essential for:
- Sending live updates about aircraft positions, speeds, and altitudes.
- Notifying users when a particular flight takes off, changes altitude, or lands.
- Displaying dynamic flight paths or updating flight details without requiring the user to refresh their browser.
WebSockets ensure that your flight tracking application remains efficient and responsive, providing users with up-to-the-minute information on any aircraft they’re following.
Creating a User-Friendly Interface
Once the server-side components are set up, the next step is to build a web-based front-end where users can interact with your flight tracking system. A simple interface might include a list of flights, showing details like the flight number, origin, destination, and current altitude. More advanced interfaces could incorporate maps that display real-time flight paths, using tools like Google Maps or Leaflet to visualize the data.
In addition to displaying flight information, your interface should allow users to search for specific flights, filter results by airline or airport, and perhaps even receive notifications when certain events occur (such as when a flight is about to land).
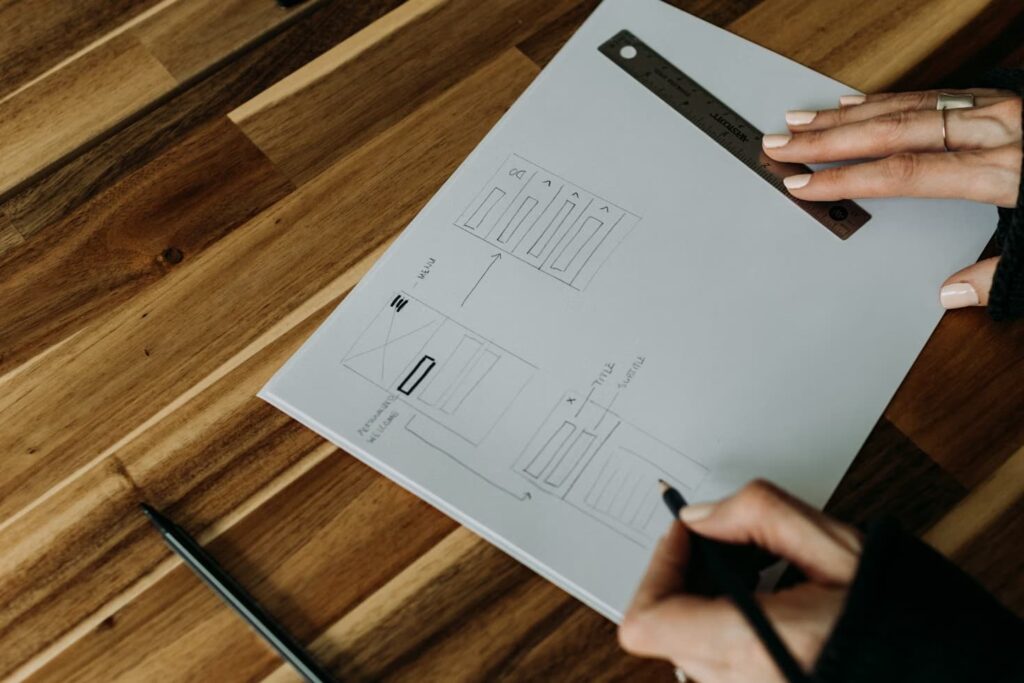
To enhance the user experience, consider:
- Integrating a map view that shows the real-time location of aircraft.
- Adding features that let users track specific flights, either by flight number or origin/destination airports.
- Displaying flight statuses (e.g., “on time,” “delayed,” “landed”) and updating them in real-time.
Flight tracking maps can be a particularly engaging feature, allowing users to see not only textual data but also visualize the flight paths of planes in real-time. This can be further enhanced with animations that show the aircraft moving across the map as updates are received from the server.
Handling Real-Time Data Load
Depending on the scope of your flight tracking project, you might need to account for performance and scalability. Handling a large number of simultaneous users or frequent requests for live flight data can place a heavy load on your server. To ensure your system remains responsive under heavy load, you should:
- Implement caching mechanisms to reduce the number of redundant requests to the external flight tracking API.
- Optimize the frequency of API requests to strike a balance between real-time accuracy and efficient use of resources.
- Scale your server as necessary, especially if you’re expecting high traffic or need to track flights globally in real-time.
By optimizing how your server handles requests and distributes updates to users, you can ensure that your flight tracking application remains performant even as the number of connected users grows.